Week 6 (Threads)
You must save your registers because someone else may use the registersCallee (vs Caller) Functions
----------
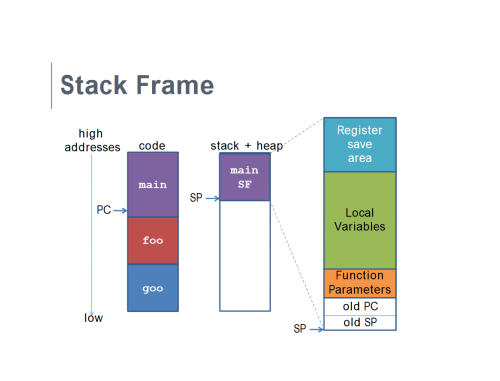
Bottom is low, Top is high (in terms of address)
For loop is usually better than recursive functions because the stack frame size can be determined for the for loop and not the recursive function (except when for loop uses iteration count that is not determined at compile time)
Stack pointer is a value stored in stack pointer register (%esp) (32 bit stack pointer register) pointing to the beginning of the stack frame
A program counter is a value stored in the program counter register (%eip) (32 bit program counter register) pointing to a point in the text
----------
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
if have multiple args, then the args are in a struct of which the pointer is put into pthread_create
it returns 0; on error returns an error number and the contents of the thread are undefined.
int pthread_join(pthread_t thread, void **retval);
int pthread_detach(pthread_t thread);
The pthread_detach() function marks the thread identified by thread
as detached. When a detached thread terminates, its resources are
automatically released back to the system without the need for
another thread to join with the terminated thread.
On success, pthread_detach() returns 0; on error, it returns an error
number.
pthread_cancel is kinda like kill (sends a cancellation request to a thread)
After a canceled thread has terminated, a join with that thread using
pthread_join(3) obtains PTHREAD_CANCELED as the thread's exit status.
(Joining with a thread is the only way to know that cancellation has
completed.)
void pthread_exit(void *retval);
The pthread_exit() function terminates the calling thread and returns
a value via retval that (if the thread is joinable) is available to
another thread in the same process that calls pthread_join(3).
SO YEAH KINDA LIKE WAIT AND EXIT. DEJA VU.
----------
A nice use case of join is - say for example the main() function/thread creates a thread and doesn't wait ( using join ) for the created thread to complete and simply exits, then the newly created thread will also stop!
You should call detach if you're not going to wait for the thread to complete with join but the thread instead will just keep running until it's done and then terminate without having the main thread waiting for it specifically.
detach basically will release the resources needed to be able to implement join.
----------
Both windows and linux thread calls are gonna be tested but linux calls are gonna have more emphasis.